Enhancing Text Automation with AI Model Stacking Across Platforms
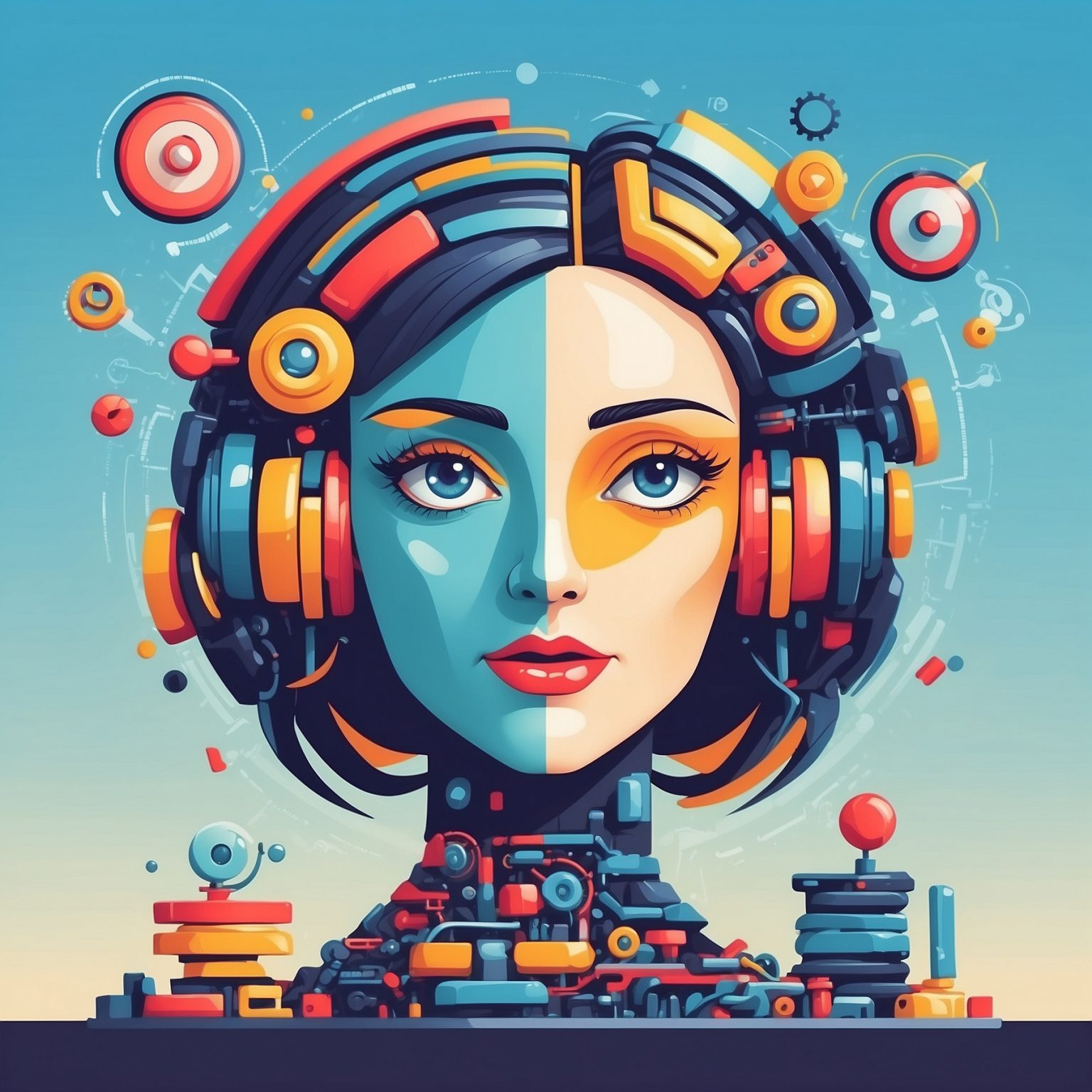
In recent years, artificial intelligence (AI) has dramatically expanded its role in automating text creation, providing tools for drafting, summarizing, translating, and creating content with minimal human input. A powerful new approach to these capabilities involves AI model stacking, or the integration of multiple AI models to create more sophisticated, reliable, and adaptable text automation workflows. Model stacking can harness strengths across different platforms—combining models like OpenAI’s GPT-4 with Anthropic’s Claude 3.5—to create versatile text automation systems tailored to specific use cases.
Understanding AI Model Stacking
Model stacking refers to the strategic use of multiple AI models, either in sequence or parallel, to leverage their combined abilities. By stacking AI models, developers can design workflows that are better suited to complex tasks that a single model might struggle to complete alone. For instance, using OpenAI’s GPT-4 for its text generation and Claude 3.5’s “computer use” feature for task automation can provide a robust approach to text creation and data processing.
One common approach is sequential stacking, where outputs from one model feed directly into another. For example, a large language model (LLM) like GPT-4 might generate draft content, which is then fed to Claude 3.5 for refining, formatting, or extracting key insights. This allows each model to play to its strengths: GPT-4 for nuanced text generation and Claude 3.5 for analytical structuring and formatting.
Leveraging Multi-Model Platforms: The Claude 3.5 Example
Anthropic’s recent updates to the Claude model, such as Claude 3.5 Haiku and Claude 3.5 Sonnet, introduce robust new capabilities tailored to specific uses. Notably, Claude 3.5’s “computer use” feature enables the model to perform computer-like interactions, such as screen navigation, cursor control, and keyboard input. This allows Claude 3.5 to automate repetitive or multistep tasks across web applications, which is especially valuable for automating data entry, research, and workflow tasks that involve multiple steps. Combined with the text generation capabilities of models like GPT-4, Claude 3.5 can process, structure, and enhance content across formats and platforms.
For instance, Claude 3.5 Sonnet, optimized for coding and tool use, can be integrated as a support model to validate and test content generated by another AI. Developers have successfully applied Claude 3.5 Sonnet’s computer-use feature to facilitate end-to-end content workflows. In these scenarios, Claude not only manages the logic of text structure but can also execute specific web-based actions, expanding the boundaries of what’s possible in automated text workflows.
Use Cases for AI Model Stacking in Text Automation
AI model stacking can enhance a wide array of tasks in text automation:
- Content Creation and Curation: For content-heavy industries, model stacking can accelerate writing, summarizing, and organizing content. GPT-4, with its broad understanding and creativity, can generate high-quality content drafts. Claude 3.5 can then analyze the generated text, format it to meet specific guidelines, and use its computer skills to post content to web platforms or fill in forms based on external data sources.
- Data-Driven Content Automation: Many content types, like reports and whitepapers, require not only text generation but also data analysis. Stacking OpenAI’s models with Anthropic’s Claude can help synthesize data and generate text around that data. For instance, Claude 3.5 Haiku, with its fast processing speed, can first parse and analyze a large dataset and pass structured insights to GPT-4 to form coherent narratives or summarize findings.
- Automated Research and Report Generation: In industries like finance and legal services, generating reports from multiple data sources can be labor-intensive. Model stacking provides a scalable solution. For example, a workflow could involve Claude 3.5 for gathering data from online sources, moving through web pages, and taking structured notes, which are then passed to GPT-4 to format into cohesive, professionally structured reports.
- Customer Support and Interactive Text Automation: Customer service often requires AI systems to handle nuanced interactions and respond to customer inquiries with precise answers. By stacking models, businesses can train GPT-4 to handle the conversational flow and pass complex queries to Claude for more technical, automated responses.
- Multilingual Content Localization: Combining models that are strong in language generation with those optimized for cultural nuance or specific language structures (e.g., a Claude model optimized for concise language) enables AI to generate content that resonates across multiple languages.
Best Practices for Implementing Model Stacking
To create effective model-stacking workflows, consider the following strategies:
- Identify Task Dependencies: Clearly define where one model’s output should feed into another. This can involve structuring workflows where each model performs specific parts of the task, enhancing consistency and speed. For example, using GPT-4 for broad text generation and Claude 3.5 to filter or structure outputs ensures each model contributes based on its unique strengths.
- Prioritize Model Strengths: Leverage each model’s primary competencies to maximize efficiency. Models like Claude 3.5 Sonnet are optimized for computer interactions and task completion, making them suitable for UI navigation or filling out forms. In contrast, models like GPT-4 excel in rich, creative text generation.
- Safety and Monitoring: Model stacking increases complexity, which makes monitoring crucial. Since each model may contribute to decision-making, it’s vital to track the impact of each stage in the workflow. For Claude 3.5, Anthropic has introduced enhanced safety checks, and implementing these alongside OpenAI’s safety protocols can further protect your workflow from undesirable outputs.
- Iterate and Test: Testing is essential for stacking, particularly when combining models from different providers. Regular testing helps identify points of friction and allows for optimization, which can prevent workflow disruptions in production environments.
Looking Ahead: The Future of Model Stacking in AI Text Automation
As the capabilities of models like Claude and GPT continue to evolve, model stacking will unlock even greater potential for text automation and beyond. The integration of computer use capabilities from Claude is one example of how AI is moving toward greater functional autonomy, which will likely influence future developments in model interoperability and AI coordination.
Looking forward, model stacking may soon integrate specialized models for image and multimedia analysis, allowing for even richer content generation workflows. As more companies adopt these methods, a collaborative approach across AI models from diverse providers will become the norm, leading to faster, safer, and more versatile text automation solutions.
AI model stacking represents a pivotal shift, allowing developers to optimize each model’s capabilities and create automation workflows tailored to specific, often complex tasks. With this approach, the future of text automation is poised to become not only more efficient but also remarkably adaptive to the diverse needs of businesses and industries.
Some Model Stacking Code Examples
Using Python:
import openai
# Replace with your OpenAI API key
openai.api_key = "your_openai_api_key_here"
def generate_blog_post(topic: str, max_tokens: int = 1000):
"""
Calls OpenAI's ChatGPT to generate a blog post draft on a specified topic.
Args:
topic (str): The topic for the blog post.
max_tokens (int): The maximum number of tokens (words) for the response.
Returns:
str: A draft of a blog post about the specified topic.
"""
prompt = f"Write a detailed blog post draft about '{topic}' focusing on recent advancements, applications, and future potential of Artificial Intelligence."
try:
# Call OpenAI's ChatGPT model
response = openai.Completion.create(
engine="text-davinci-003", # Use "gpt-4" if you have access to it
prompt=prompt,
max_tokens=max_tokens,
temperature=0.7 # Adjust creativity; 0.7 is a balanced level
)
# Extract the response text
blog_post_draft = response.choices[0].text.strip()
return blog_post_draft
except Exception as e:
print(f"An error occurred: {e}")
return ""
# Example usage
topic = "The Impact of Artificial Intelligence on Modern Education"
blog_post = generate_blog_post(topic)
print(blog_post)
Then, modify your code to use Anthopic’s API to revise the original creation from OpenAI like this:
import openai
import anthropic
# Replace these with your OpenAI and Anthropic API keys
openai.api_key = "your_openai_api_key_here"
anthropic_client = anthropic.Client(api_key="your_anthropic_api_key_here")
def generate_blog_post_openai(topic: str, max_tokens: int = 1000):
"""
Calls OpenAI's ChatGPT to generate a blog post draft on a specified topic.
Args:
topic (str): The topic for the blog post.
max_tokens (int): The maximum number of tokens (words) for the response.
Returns:
str: A draft of a blog post about the specified topic.
"""
prompt = f"Write a detailed blog post draft about '{topic}' focusing on recent advancements, applications, and future potential of Artificial Intelligence."
try:
# Call OpenAI's ChatGPT model
response = openai.Completion.create(
engine="text-davinci-003", # Use "gpt-4" if you have access to it
prompt=prompt,
max_tokens=max_tokens,
temperature=0.7
)
# Extract the response text
blog_post_draft = response.choices[0].text.strip()
return blog_post_draft
except Exception as e:
print(f"An error occurred with OpenAI: {e}")
return ""
def revise_blog_post_anthropic(text: str):
"""
Calls Anthropic's Claude model to revise a blog post draft created by OpenAI.
Args:
text (str): The initial blog post draft text to be revised.
Returns:
str: A revised version of the blog post.
"""
anthropic_prompt = f"{anthropic.HUMAN_PROMPT} Please revise and improve the following blog post to make it clearer, more engaging, and professional:\n\n{text}\n\n{anthropic.AI_PROMPT}"
try:
# Call Anthropic's Claude model
response = anthropic_client.completions.create(
model="claude-1", # Or "claude-2" depending on access level
prompt=anthropic_prompt,
max_tokens_to_sample=1000,
temperature=0.7
)
# Extract the revised text from Claude's response
revised_blog_post = response["completion"].strip()
return revised_blog_post
except Exception as e:
print(f"An error occurred with Anthropic: {e}")
return ""
# Example usage
topic = "The Impact of Artificial Intelligence on Modern Education"
# Step 1: Generate the initial draft with OpenAI
initial_blog_post = generate_blog_post_openai(topic)
print("Initial Draft from OpenAI:\n", initial_blog_post)
# Step 2: Revise the draft with Anthropic's Claude
revised_blog_post = revise_blog_post_anthropic(initial_blog_post)
print("\nRevised Draft from Anthropic:\n", revised_blog_post)
Explanation of the Code:
- Generate Initial Draft: The
generate_blog_post_openai
function uses OpenAI’s API to generate the first draft. - Revise Draft with Claude: The
revise_blog_post_anthropic
function takes the draft and uses Anthropic’s Claude model to enhance it. This function sends a prompt to Claude asking for clarity, engagement, and a more professional tone. - Prompt Formatting: The Anthropic API uses distinct markers (
anthropic.HUMAN_PROMPT
andanthropic.AI_PROMPT
) to denote human and AI input.
Key Parameters:
- Model Selection: Replace
"text-davinci-003"
and"claude-1"
as needed based on available models and desired complexity. Both of these models are no longer available, I seem to recall. - Temperature and Max Tokens: Adjust for more creativity or to set a limit on response length.
Ensure both API keys are kept secure and replace the placeholders with actual keys for production use.